TeamDrive Http Api¶
Overview¶
The TeamDrive Agent offers a JSON interface via HTTP, which allows you to operate
the TeamDrive Agent without using the GUI. The JSON interface can be activated by specifying the teamdrived --http-api-port
option.
The command line application TeamDriveApi.py is located in the TeamDrive Agent installation directory (e.g. C:\Program Files (x86)\TeamDrive 3 Agent). Running this file allows you to use the TeamDrive API directly. See Options for a list of Options and Settings.
If you would like to use the TeamDrive HTTP API without Python, HTTP GET requests can be made directly through TeamDrive Agent. The URL will need to be constructed as follows:
http://<host>/api/<command>?<parameters>
For example:
http://[::1]:45454/api/getSpace?id=1
The result is a JSON document.
Requirements¶
In order to run use the interface, you will need the these requirements:
- TeamDrive Desktop Client or TeamDrive Agent
The Python module and Command line interface
requires
- Python in your PATH variable.
- Recommended: docopt for Python.
TeamDriveApi.TeamDriveApi.sse()
requires Python 3.
Security Considerations¶
Communicating with the TeamDrive Http Api contains sensitive information, for example user names, passwords and file data. There are mechanisms to provide authentication and privacy.
Restricting the availability of the Api¶
By restricting the api to the local host, any attempt to access the api from another host will fail:
http-api-port=127.0.0.1:<port>
Although, this does not prevent the usage of the api from another user of this system.
Using a local socket or a named pipe¶
One can further reduce the availability by specifying the type of the api like this:
http-api-type=socket
http-api-port=<name>
<name> can be a user defined string. The Unix socket file will be placed in /tmp on Mac OS X and on Linux.
Using HTTPS for communication with the TeamDrive Agent¶
HTTPS can be enabled by setting type to ssl like this:
http-api-type=ssl
http-api-port=443
It is recommended to use port 443 for communication with browsers, because using a non-standard port for HTTPS
is considered insecure. Please also have a look at the teamdrived --http-api-certificate
and teamdrived --http-api-private-key
settings.
Note
The HTTPS server that is used by the TeamDrie Agent is based on the Qt framework, which supports, according to the
documentation, the following protocols:
SSLv2
, SSLv3
, TLSv1.0
, TLSv1.1
and TLSv1.2
.
Authentication¶
In order to prevent unauthorized users from accessing the TeamDrive Http Api, an authentication is required to access the api. There are two ways to authenticate clients:
- Statefull Session Cookies
- Stateless Authorization Header
Session Cookies¶
Session Cookies are mainly used to authenticate Web Browsers. Using a session requires a call to
TeamDriveApi.TeamDriveApi.login()
to retrieve a cookie from the server. TeamDriveApi.TeamDriveApi.getLoginInformation()
can be used to determine if a login is required.
Login with the Api using Cookies should follow this protocol:
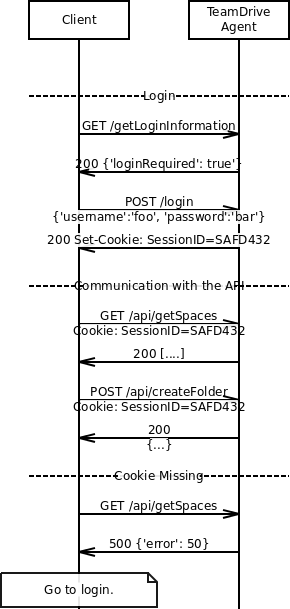
In the case of a missing Cookie, a TeamDriveApi.TeamDriveException.Error_Missing_Session
is returned
Authorization Header¶
This stateless alternative to Cookies uses HTTP Basic Authentication. Every request to the Api requires an Authorization Header following RFC 2617.
Currently, The Agent does not answer requests without Authentication Header appropriately. It is expected, that clients send the Authorization Header unconditionally.
Interface Overview¶
TeamDrive provides two API endpoints:
- The
/api
endpoint provides meta data about all elements of TeamDrive - The
/files
endpoint provides an interface for reading and writing the file content.
Communicating with the /api endpoint¶
This is the reference documentation of the TeamDrive Client API. As a convenience, there is a Python module that provides accessor functions for each API call, which is also usable as a command line interface. Please have a look at all possible usages.
Example using curl:
$ curl '127.0.0.1:45454/api/getLoginInformation'
{ "isLoginRequired" : true }
Example using the Python module:
>>> from TeamDriveApi import TeamDriveApi
>>> api = TeamDriveApi("127.0.0.1:45454")
>>> map(api.getSpace, api.getSpaceIds())
[{.....}]
Example using the command line:
./TeamDriveApi.py '127.0.0.1:45454' getLoginInformation
{
"username": "My Username",
"apiUrl": "/",
"isLoginRequired": false
}
Example Session (Initial Login):
# Start TeamDrive:
exec /opt/teamdrive/teamdrived
# Wait for it:
sleep 5
# Log-in
./TeamDriveApi.py '127.0.0.1:45454' --user myUser --pass myPass login
# Create a new Space
./TeamDriveApi.py '127.0.0.1:45454' --user myUser --pass myPass createSpace
# Call Functions
./TeamDriveApi.py '127.0.0.1:45454' --user myUser --pass myPass createFolder
# Quit TeamDrive without logout
./TeamDriveApi.py '127.0.0.1:45454' --user myUser --pass myPass quit False
Example Session:
exec /opt/teamdrive/teamdrived
sleep 5
# No need to login now
./TeamDriveApi.py '127.0.0.1:45454' --user myUser --pass myPass createFolder
# Quit TeamDrive without logout
./TeamDriveApi.py '127.0.0.1:45454' --user myUser --pass myPass quit False
Example Session (After previous Logout:
# If the previous session ended with a logout:
./TeamDriveApi.py '127.0.0.1:45454' --user myUser --pass myPass quit True
# The next call should start with a login:
exec /opt/teamdrive/teamdrived
sleep 5
./TeamDriveApi.py '127.0.0.1:45454' --user myUser --pass myPass login
./TeamDriveApi.py '127.0.0.1:45454' --user myUser --pass myPass createFolder
See also
TeamDriveApi.TeamDriveException
for Errors returned by the API.
See also
Chapter Authentication regarding Authentication.
Communicating with the /files endpoint¶
See TeamDriveApi.TeamDriveApi.putFile()
for Details.
Passing Parameters to the API¶
The format of parameters depend on the HTTP method:
- Parameters for GET-requests should be passed by a query string in the url. For example
curl --user myTeamDriveUser http://127.0.0.1:45454/api/getSpace?id=310
- Parameters to POST-requests can either be a JSON object or data encoded using
application/x-www-form-urlencoded
.
Interface Description¶
TeamDriveApi.TeamDriveApi¶
-
class
TeamDriveApi.
TeamDriveApi
(server='[::1]:45454', username='', password='')¶ Return type: Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
-
about
()¶ Returns information about TeamDrive.
Returns: { "version": "4.0.12 (Build: 1294)", }
Changed in version 4.1.1: no longer returns license infos. call
getLicense
instead.Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
addAddressbook
(name)¶ Adds a new Address book entry. TeamDrive will lookup that name on the RegServer. If that fails, an exception is thrown. You cannot add email addresses.
Using this function requires the HTTP POST method.
Parameters: name (str) – the new Addressbook’s name.
Returns: the id of the new entry.
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – user is unknown.
- ValueError – no JSON returned. Wrong URL?
-
addComment
(fileId, comment, notifyMembers)¶ Attaches the comment to the file.
Using this function requires the HTTP POST method.
See also
getComments
,getSpaceComments
,addSpaceComment
andremoveComment
New in version 4.2.1.
Parameters: notifyMembers (bool) – Send notification to all members (default is false) New in version 4.1.1.
Parameters: - fileId (int) – The corresponding id of the file
- comment (str) – The content of the new comment
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Adding this comment failed.
- ValueError – no JSON returned. Wrong URL?
-
addServer
(url, name, username, password, path, type)¶ Register a WebDAV or TDPS server for use by the TeamDrive client.
Parameters: - url (str) – Url of the server
- name (str) – Name of the server in TeamDrive
- username (str) – WebDAV only: username for the server
- password (str) – WebDAV only: password for the server
- path (str) – WebDAV only: path to the server
- type (str) – ‘TDPS’ or ‘WebDAV’
Return type: dict
-
addSpaceComment
(spaceId, comment, notifyMembers)¶ Adds this comment to the Space.
Using this function requires the HTTP POST method.
See also
New in version 4.2.1.
Parameters: notifyMembers (bool) – Send notification to all members (default is false) New in version 4.1.1.
Parameters: - spaceId (int) – The Space id.
- comment (str) – The content of the new comment
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Adding this comment failed
- ValueError – no JSON returned. Wrong URL?
-
cancelProxyAuthentication
()¶ Cancel Proxy-Authentication process.
Return type: dict
-
clearProfilePicture
()¶ Clears the profile picture of the current user.
See also
getProfilePicture
to retrieve profile pictures andsetProfilePicture
New in version 4.1.1.
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
commitCurrentSnapshot
(spaceId)¶ Commit the snapshot that is currently being previewed.
The process for restoring a Space to a snapshot is as follows:
‘preview’ a snapshot by calling the
joinSpace
function with the ‘snapshotId’ parameter- The Space is reverted locally to the state stored in the snapshot, in read-only mode
- The user can then decide whether they want to commit this change.
‘commit’ the change by calling
commitCurrentSnapshot
- The Space is reverted on the server (this affects not only files but also the members in the Space, member permissions, etc.)
While in preview-mode, you can also call
joinSpace
again with a different snapshot ID to preview another snapshot, or without a snapshot ID to cancel the rollback and restore the Space to its usual state.Using this function requires the HTTP POST method.
New in version 4.3.2.
Parameters: spaceId (int) – The Space id. Return type: bool
-
confirmSuperPinExport
()¶ This function marks the super-PIN/account recovery data as “exported” - ie. the user has stored it in some safe place. With the recovery data marked as “exported”, TeamDrive will stop reminding the user to take care of this task.
Return type: dict
-
createBackup
()¶ Create a backup in the local file system. To create a backup and download it, send a GET request to ‘/backup’. To import a TeamDrive backup file (‘.tdbk’), send it to ‘/backup’ via a PUT request.
Returns: A dict where the key “path” points to the file in the local filesystem. New in version 4.3.1.
Return type: dict
-
createFolder
(spaceId, filePath)¶ Creates a new folder in that Space
Example:
$ ./TeamDriveApi.py '127.0.0.1:45454' createFolder 3 '/my new folder' False
{ "result": true, "file": { "isDir": true, "name": "my new folder", "currentVersionId": 0, "spaceId": 3, "isInFileSystem": false, "path": "/", "id": 756, "permissions": "rwxr-xr-x" } }
See also
getFullFolderContent
orgetFolderContent
for getting the content of this folderNew in version 4.0.
Returns: A dict containing a File object
Parameters: - spaceId (int) – The Id of the Space
- filePath (str) – The full folder path.
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
createInbox
(folderId, expiryTime=None, password=None, description=None, maxFileCount=None, maxFileCountInterval=None, maxFileSize=None, maxSize=None)¶ Convert the given folder into an inbox, allowing anybody with the link to the inbox to upload files into the folder (the inbox service needs to already be set up in order to enable this functionality).
Parameters: - folderId (int) – The id of the folder to convert to an inbox
- expiryTime (str) – Optional date on which the inbox-access to this folder will automatically be disabled again, for example “2020-09-30T10:52:15.762Z”.
- password (str) – If set, the password people need to enter before uploading to the inbox.
- description (str) – Optional description for the inbox
- maxFileCount (int) – Optional maximum number of files that can be uploaded to the inbox in the interval specified by
maxFileCountInterval
(Only has an effect ifmaxFileCountInterval
is not set to “Unlimited”) - maxFileCountInterval (str) – One of “Unlimited”, “Infinite”, “Day”, “Week”, “Month”, “Quarter”, “HalfYear”, “Year”. For example, if maxFileCount is set to 10, and this parameter is set to “Week”, at most 10 files may be uploaded to the inbox per week. If this parameter were set to “infinite” instead, then the inbox would only accept at most 10 files in total. The default is “Unlimited”.
- maxFileSize (int) – Optional limit on the size of individual uploaded files, in bytes.
- maxSize (int) – Optional limit on the total file size of all files uploaded to the inbox, in bytes.
Return type: dict
-
createSnapshot
(spaceId)¶ Create a snapshot that can later be used to restore the Space (see
commitCurrentSnapshot
)Using this function requires the HTTP POST method.
New in version 4.3.2.
Parameters: spaceId (int) – The Space id. Return type: bool
-
createSpace
(spaceName, disableFileSystem=None, spacePath=None, importExisting=None, serverId=None, inviteOwnDevices=None, versionCount=None, webAccess=None, dataRetentionPeriod=None, dataRetentionAutoTrash=None, dataRetentionDeleteGuard=None, defaultJoinMode=None, mountInVirtualFS=None)¶ Creates a new Space with the given name.
To convert an existing folder into a TeamDrive Space, you need to set the importExisting parameter to True.
In case the url is called directly, the default for disableFileSystem is False and the spacePath is mandatory.
“Data Retention” Spaces
Spefifying the
dataRetentionPeriod
parameter creates a special “Data Retention” Space. Any file in the Space will not be deletable by anyone except the Space Administrator until the file is older than the specified retention period. This can be used to satisfy legal requirements that documents be retained for a minimum length of time.Using this function requires the HTTP POST method.
Throws
TeamDriveException
, if the call fails.returns: A dict containig the Id and a Space. Parameters: - spaceName (str) – The Name of the new Space
- disableFileSystem (bool) – This will disable the synchronization into the file system. TeamDrive will only synchronize meta-data if this is True.
- spacePath (str) – File Path, in which the new Space will be created.
- importExisting (bool) – Tells the TeamDrive Agent to import an existing directory.
- serverId (int | None) – Hostserver to use
- inviteOwnDevices (bool | None) – Whether to automatically invite your other teamdrive installations
- versionCount (int | None) – Maximum number of versions of each file that will be kept
- webAccess (str | None) –
Whether this Space may be accessed from the web (one of “true”, “false”, or “default”)
New in version 4.3.2.
- dataRetentionPeriod (int | None) –
Minimum length of time (in months) before files can be deleted
New in version 4.6.3.
- dataRetentionDeleteGuard (bool | None) –
- By default, files can still be deleted within the “data retention” period after an additional password check. If this parameter
- is set to
true
, such deletions will be prevented as well.
New in version 4.6.3.
- dataRetentionAutoTrash – Whether to automatically move files to the trash at the end of the data retention period
New in version 4.6.3.
Parameters: defaultjoinMode – How members should join this Space by default (ie. the option that people who are invited to the Space will see auto-selected). One of: * default -> The default settings of the invited user will be shown as auto-selected * offline-available -> By default, the Space will be joined in offline-available mode (ie. all files downloaded locally) * non-offline-available * virtual -> Space will be mounted as a folder in the virtual file system (where possible) New in version 4.6.7.
Parameters: mountInVirtualFS – Whether to mount the Space as a folder in the virtual file system. New in version 4.6.7.
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Space creation failed.
- ValueError – no JSON returned. Wrong URL?
-
deleteFileFromTrash
(fileId)¶ Deletes a file from Trash by physically deleting the file from the server. This call fails, if you do not have the rights to delete files, it is a directory or the file is not in the trash.
Using this function requires the HTTP POST method.
Example:
>>> api = TeamDriveApi() >>> for inTrash in api.getFullFolderContent(1, "/", True): >>> api.deleteFileFromTrash(inTrash["id"])
Throws
TeamDriveException
, if the call fails.See also
New in version 4.0.
Parameters: fileId (int) – The Id of the Space
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Failed to queue command.
- ValueError – no JSON returned. Wrong URL?
-
deleteInbox
(id)¶ Delete the given inbox (ie. remove upload-access to the folder via the inbox URL, neither the folder itself nor any files in it will be affected).
Parameters: id (int) – The id of the Inbox Return type: dict
-
deleteServer
(id)¶ Delete a WebDAV or TDPS server.
Parameters: id (int) – Id of the server Return type: dict
-
deleteSnapshot
(spaceId, snapshotId)¶ Delete snapshots for this Space based on the given
snapshotId
Warning
This function deletes all snapshots up to and including the one specified by
snapshotId
Using this function requires the HTTP POST method.
New in version 4.5.0.
Parameters: - spaceId (int) – The Space id.
- snapshotId (int) – The snapshot id.
Return type: bool
-
deleteSpace
(id, delInFs, delOnServer, delInDB)¶ Deletes a Space.
Using this function requires the HTTP POST method.
Throws
TeamDriveException
, if the call fails.Parameters: - id (int) – The Id of the Space
- delInFs (bool) – Delete the Space in the File System
- delOnServer (bool) – Deletes the Space on the server. (requires Administrator rights)
- delInDB (bool) – Deletes the Space in the local Database
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Deletion failed
- ValueError – no JSON returned. Wrong URL?
-
deleteVersion
(versionId)¶ Deletes a file version finally from the server. This call fails, may may not have the rights to delete files,
Using this function requires the HTTP POST method.
Throws
TeamDriveException
, if the call fails.See also
New in version 4.1.4.
Parameters: versionId (int) – The Id of the version
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Failed to queue command.
- ValueError – no JSON returned. Wrong URL?
-
editServer
(id, url, name, username, password, path)¶ Edit a WebDAV or TDPS server.
Parameters: - id (int) – Id of the server
- url (str) – Url of the server
- name (str) – Name of the server in TeamDrive
- username (str) – WebDAV only: username for the server
- password (str) – WebDAV only: password for the server
- path (str) – WebDAV only: path to the server
Return type: dict
-
emptyTrash
(spaceId)¶ Permanently delete all trashed files for the given Space.
Note
Depending on the number of files / file versions, the contents of the trash may not immediately disappear after this function returns
Using this function requires the HTTP POST method.
New in version 4.5.3.
Parameters: spaceId (int) – The Space id. Return type: dict
-
exportRecoveryData
()¶ Export recovery data (the “super PIN” and a recovery-URL).
Return type: dict
-
findFiles
(spaceIds=None, path='/', search=None, recursive=False, filters=None, sortBy='name', start=0, limit=-1)¶ This function can be used to create a paged list of files in all Spaces, a single Space, or a single folder.
The result is returned like this:
{ totalFiles: 123, files: [...] }
totalFiles
is the number of files that were matched,files
is a subset of the matched files based on thestart
andlimit
parameters. SeegetFile
for the format of the individual file objects.The parameter
filter
is a comma-seperated list of strings indicating special filters. Valid values are:conflicted
: Show only files that have name- or version conflictsdirectory
: Show only folderstrashed
: Only files that are in the trashinvalidPath
: Only files that have an invalid path or name for the OS that the agent is running onofflineAvailable
: Show only files that have been set to “offline-available”
The above filters can also be prefixed with a ”!” to invert them, eg.
!trashed
to show only files that are not in the trashAdditionally, one of the following can be included in the filter list (these values can’t be prefixed with ”!”):
unconfirmed
: Show only files where the latest version has not been processed by TeamDrive yetcommented
: Show only files that have commentspublished
: Show only published files
The returned list is sorted according to the
sortBy
parameter, which contains a list of file properties that are considered in order (eg. specify[isDir, name]
to sort by name, but list directories first). Valid values in the list are:isDir
: List directories firstname
: Sort by file nameadded
: sort by the time this file was added to the Spacecreated
: Sort by date of file creationsize
: Sort by size (directories considered as having a size of 0)modified
: Sort by last-modified date (directories are sorted as though the date was 0)
All of the sort-types are in ascending order by default, and can be inverted by prefixing them with ”!”, eg.
!size
to list in order of descending size.New in version 4.5.1.
Parameters: - spaceIds (list[int]) – The IDs of the Spaces to search (default if not specified: search all Spaces)
- path (str) – The path in the Space(s) under which to search for files. (Default is ‘/’)
- search (str) – If set, files will be filtered by matching the file name against this parameter
- recursive (bool) – Whether to recursively search subfolders of the given path (
False
by default) - filters (list[str]) – A comma-separated list of special filters. See the descriprion of this function for details
- sortBy (list[str]) – A list of file properties to sort by, see the description of this function for details (default is
name
) - start (int) – The first row of the matched set of files to return (0-indexed, the default if not specified is
0
) - limit (int) – Maximum number of rows to return, or
-1
to return all rows (default is-1
)
Returns: A dict with the number of files matched, and the subset of those files specified by
start
andlimit
Return type: dict
-
findUser
(query)¶ Returns information on a TeamDrive user, queried either by name or email address (the TeamDrive user must already be in the local address book).
Parameters: query (str) – Name or Email Return type: dict
-
getActivities
(spaceIds=None, fromDate=None, toDate=None, searchTerm=None, typeFilter=None, userFilter=None)¶ Returns a list of all activities for the given space list within the date period or for the last 30 days. If
hasDetails
istrue
, a call togetActivityDetails
with the given activityId will return a list of details.Possible activity types are:
userInvited
userJoined
userLeft
userKicked
commentCreated
commentDeleted
spaceCommentCreated
spaceCommentDeleted
versionAdded
versionDeleted
versionPublished
versionUnpublished
versionExpired
Changed in version 4.2.0.
Added new activity types:
userRightsChanged
userProfileUserNameChanged
userProfileEmailChanged
userProfilePhoneChanged
userProfileMobileChanged
userProfileNotesChanged
userProfilePictureChanged
userProfileInitialsChanged
userLicenseChanged
userRegEmailChanged
spaceMaxVersionsOnServerChanged
spaceMetaDataChanged
spaceMetaDataDeleted
spaceDeletedOnServer
serverAccessSent
serverAccessFailedToSend
serverAccessReceived
serverAccessDeleted
invitationSent
invitationFailed
invitationDeclined
invitationReceived
fileAdded
fileRenamed
fileMoved
fileTrashed
fileUntrashed
fileDeleted
fileChanged
fileCurrentVersionChanged
folderAdded
folderRenamed
folderMoved
folderTrashed
folderUntrashed
folderDeleted
folderChanged
fileNameConflict
fileNameConflictResolved
filePathInvalid
filePathInvalidResolved
versionPublishMetaChanged
versionConflict
versionConflictResolved
versionExported
emailNotificationSent
emailNotificationFailedToSend
workingDirFull
runningOutOfDiskSpace
noDiskFreeSpace
automaticTrashCleanupChanged
temporaryError
See also
New in version 4.1.1.
Returns: A list of activities.
Return type: list[dict]
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
Changed in version 4.2.0.
Parameters: - spaceIds (str) – A list of space IDs. If empty, all Spaces will be used.
- fromDate (str) – ISO 8601 extended format: either YYYY-MM-DD for dates or YYYY-MM-DDTHH:mm:ss, YYYY-MM-DDTHH:mm:ssTZD (e.g., 1997-07-16T19:20:30+01:00) for combined dates and times. If empty the last 30 days will be returned.
- toDate (str) – ISO 8601 extended format: either YYYY-MM-DD for dates or YYYY-MM-DDTHH:mm:ss, YYYY-MM-DDTHH:mm:ssTZD (e.g., 1997-07-16T19:20:30+01:00) for combined dates and times.
New in version 4.3.2.
Parameters: - searchTerm (str) – Search for activities that match the given string
- typeFilter (str) – Comma-separated combination of:
conflictAndError
,invitationAndMember
,fileAndVersion
,comment
,publish
,all
- userFilter (str) – one of:
myUser
,otherUsers
,all
-
getActivityDetails
(activityId)¶ Returns a list of all activity details for an activity.
See also
New in version 4.1.1.
Parameters: activityId (str) – The activityId as returned by
getActivities
Returns: A list of activityDetails.
Return type: list[dict]
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getActivityMetas
(activityId)¶ Returns a list of all activity meta information for an activity.
See also
New in version 4.2.1.
Parameters: activityId (str) – The activityId as returned by
getActivities
Returns: A list of activityMeta.
Return type: list[dict]
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getAddressbook
(id)¶ Returns information about a given Addressbook Id.
See also
getFullAddressbook
for an exampleParameters: id (int) – the Address Id.
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getAddressbookByName
(addressName)¶ This is convenience wrapper of the Python module that is not part of the official API.
Returns an Addressbook entry by a given user name. Throws a TeamDriveException, if there is no Space with this name.
Parameters: addressName (str) – The Name.
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveCallFailed – There is no Space with this name
- ValueError – no JSON returned. Wrong URL?
-
getAddressbookIds
()¶ Returns all knows Addressbook Ids.
Return type: list[int]
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getComments
(fileId)¶ Returns a list of all comments that are attached to a specific file in a space.
Example
>>> api = TeamDriveApi() >>> api.getComments(4183)
Returns: [ { "creator": "test", "creatorAddressId": 1, "text": "file baz", "created": "2015-09-01T15:15:31Z", "commentId": 16, "initials": "te" }, { "creator": "test", "creatorAddressId": 1, "deleted": true, "created": "2015-09-01T15:15:28Z", "commentId": 15, "initials": "te" } ]
See also
getSpaceComments
,addComment
,addSpaceComment
andremoveComment
New in version 4.1.1.
Parameters: fileId (int) – The corresponding id of the file
Returns: A list of comments.
Return type: list[dict]
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getFile
(id)¶ Returns information about the given File Id.
returns: A File object Parameters: id (int) – The File id
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getFiles
(spaceId, filePath, trashed)¶ Returns a list of files matching Space Id, file path and trashed flag.
returns: A list of File objects Parameters: - spaceId (int) – The Id of the Space
- filePath (str) – the path of the file in the Space.
- trashed (bool) – Is the file in the trash?
Return type: list[dict]
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getFolderContent
(spaceId, filePath, trashed)¶ Returns a list of file Ids in a folder of a Space.
Example:
python ./TeamDriveApi.py '127.0.0.1:45454' getFolderContent 3 / False [756,767,737,769,629,763,628,630,830,765]
Parameters: - spaceId (int) – The Id of the Space
- filePath (str) – The parent folder path.
- trashed (bool) – In Trash?
Return type: list[int]
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getFolderInfo
(spaceId, filePath, trashed)¶ Returns information about the size of a folder:
{ "files": 10, "size": 3288549 }
New in version 4.5.0.
Parameters: - spaceId (int) – The Id of the Space
- filePath (str) – The parent folder path.
- trashed (bool) – In Trash?
Return type: list[int]
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getFullAddressbook
()¶ Returns all Addressbook entries at once.
Example:
$ python ./TeamDriveApi.py '127.0.0.1:45454' getFullAddressbook
[ { "id": 1, "email": "info@teamdrive.net", "name": "My Name", "icon": "self" } ]
Note
As this function was added with TeamDrive 4, the fall back for TeamDrive 3 is to iterate over all Addressbook Ids, therefore this function is much slower.
New in version 4.0.
Return type: list[dict]
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getFullFolderContent
(spaceId, filePath, trashed)¶ Returns a list of file information in a folder of a Space.
Example:
$ python ./TeamDriveApi.py '127.0.0.1:45454' getFullFolderContent 3 / False
[ { "isDir": true, "name": "My folder", "currentVersionId": 0, "spaceId": 3, "isInFileSystem": true, "hasNameConflict": false, "hasVersionConflict": false, "path": "/", "id": 756, "permissions": "rwxr-xr-x" }, { "isDir": false, "name": "My File", "currentVersionId": 0, "spaceId": 3, "isInFileSystem": true, "hasNameConflict": false, "hasVersionConflict": false, "path": "/", "id": 767, "permissions": "rwxr-xr-x" } ]
Note
As this function was added with TeamDrive 4, the fall back for TeamDrive 3 is to iterate over getFolderContent, therefore this function is much slower.
See also
New in version 4.0.
Returns: A list of File objects
Parameters: - spaceId (int) – The Id of the Space
- filePath (str) – The parent folder path.
- trashed (bool) – In Trash?
Return type: list[dict]
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getInbox
(id)¶ Fetch information on a given Inbox.
Parameters: id (int) – The id of the Inbox Return type: dict
-
getLicense
()¶ Returns information about the current License.
Returns: { "distributor": "DOCU", "licenseKey": "DOCU-8782-5485-7266", "licenses": [ "WebDAV", "Professional" ] }
New in version 4.1.1.
See also
setLicenseKey
to set the license.Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getLoginInformation
()¶ Returns information about the current user.
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getMember
(spaceId, addressId)¶ Returns information about a member of a Space.
Example:
$ python ./TeamDriveApi.py '127.0.0.1:45454' getMember 3 1
Returns: { "status": "active", "spaceId": 3, "addressId": 1 }
Parameters: - spaceId (int) – The Id of the Space
- addressId (int) – Already known Addressbook Id.
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getProfilePicture
(id)¶ Returns the profile picture of the address.
See also
setProfilePicture
to set the profile picture of the current user.New in version 4.1.1.
Parameters: id (int) – The addressId.
Returns: The picture.
Return type: bytes | dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
-
getProxySettings
()¶ Get current proxy settings.
Return type: dict
-
getReadConfirmations
(spaceId, addressId=0, fileId=0, filter='confirmed', onlyLatest=True, fileSearch='', limit=-1, start=0)¶ Fetch “Read Confirmations” (or missing Read Confirmations) for a Space, file, folder or Space member.
The result is returned like this:
{ totalConfirmations: 123, confirmations: [ { addressId : 1, confirmed : true, // would be False if this file version hadn't been confirmed by this user fileId : 142950, name : "new.txt", path : "/", time : 1498746732698.0, totalConfirmations : 1, // # of confirmations for this file, included if onlyLatest is True versionId : 117039, versionNo : 4 }, ... ] }
New in version 4.5.1.
Parameters: - spaceId (int) –
- addressId (int) – Only get Read Confirmations for this user (optional)
- fileId (int) – Only get Read Confirmations for this file (optional)
- filter (string) – One of
confirmed
,unconfirmed
orall
to fetch Read Confirmations, missing cofirmations, or everything (defaults toconfirmed
if not set) - onlyLatest (bool) – If
False
fetch every confirmation for every version of this file. Default isTrue
: fetch only the latest confirmation per user and file - fileSearch (string) – Filter by file name based on this string
- limit (int) – Fetch at most this many confirmations (default is
-1
, ie. no limit) - start (int) – Index from which to start returning results (default is 0, use together with
limit
to implement paging)
Returns: A dict with the number of confirmations matched, and the subset of those items specified by
start
andlimit
Return type: dict
-
getServer
(id)¶ Returns information on a TeamDrive host server, a TDPS server, or a WebDAV server.
Parameters: id (int) – The Id of the Server Return type: dict
-
getServerIds
()¶ Returns a list of Host-, TDPS, and WebDav-Server ids.
Return type: list[int]
-
getServers
()¶ Returns a list of Host-, TDPS, and WebDav-Servers.
Return type: list[dict]
-
getSettings
()¶ Returns a list of settings, with each setting a dict. An incomplete list of settings can be found in the Options Section.
Example :
{ "key": "identifier", "label": "readable label", "help": "help text", "type": "string|path|integer|bool|enum|bitmask", "value": "current value", "options": ["enum option 1", "enum option 2"], "flags": [{bit: <bit flag>, label: "readable label"}, ...] }
setSetting
can be used to set a specific setting.Return type: list
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getSnapshots
(spaceId)¶ Get snapshots that can later be used to restore the Space (see
commitCurrentSnapshot
)New in version 4.3.2.
Return type: list[dict]
-
getSpace
(id)¶ Will return information about that Space.
See also
getSpaceStatistics
for statistics.Example using Python:
>>> api = TeamDriveApi() >>> map(api.getSpace, api.getSpaceIds()) {.....}
Example using curl:
curl --user myTeamDriveUser http://127.0.0.1:45454/api/getSpace?id=310 :returns: A single :ref:`space-json-data-type`.
Parameters: id (int) – The Id of the Space
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getSpaceByName
(spaceName)¶ This is convenience wrapper of the Python module that is not part of the official API.
Returns a Space by a given Space name. Throws a
TeamDriveException
, if there is no Space with this name.Parameters: spaceName (str | unicode) – The Name.
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveCallFailed – There is no Space with this name
- ValueError – no JSON returned. Wrong URL?
-
getSpaceComments
(spaceId)¶ Returns a list of all comments that are attached to the Space itself.
Example
>>> api = TeamDriveApi() >>> api.getSpaceComments(13)
Returns: [ { "creator": "test", "creatorAddressId": 1, "text": "file baz", "created": "2015-09-01T15:15:31Z", "commentId": 16, "initials": "te" }, { "creator": "test", "creatorAddressId": 1, "deleted": true, "created": "2015-09-01T15:15:28Z", "commentId": 15, "initials": "te" } ]
See also
New in version 4.1.1.
Parameters: spaceId (int) – The Space id.
Returns: A list of comments.
Return type: list[dict]
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getSpaceIds
()¶ Returns a list of all known Spaces Ids.
Example:
>>> api = TeamDriveApi() >>> api.getSpaceIds() [1,4,5,6]
Return type: list[int]
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getSpaceMemberIds
(spaceId)¶ Returns all Addressbook Ids in a given Space.
Example:
$ python ./TeamDriveApi.py '127.0.0.1:45454' getSpaceMemberIds 3 [1,2]
New in version 4.0.
Deprecated since version 4.1.1: Use
getSpaceMembers
instead.Return type: list[int]
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getSpaceMembers
(spaceId)¶ Returns all Member infos in a given Space.
New in version 4.1.
Return type: list[dict]
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getSpacePermissionLevels
()¶ Get a list of possible space permission “levels” (ie. read, read/write, admin, etc.) and their capabilities. Permission levels are returned in order of increasing “magnitude”. The reply of this call is constant and won’t change until the agent is restarted.
Returns: [ { "id": "none", "name: "None", "rights": { "memberInvite": False, "memberKick": False, "memberSetRights": False, "spaceRename": False, "spaceDeleteOnServer": False, "filePublish": False, "fileDeleteOnServer": False, "fileRename": False, "fileRestoreFromTrash": False, "fileChange": False } }, { "id": "read", "name": "Read" "rights": { ... } }, ... ]
New in version 4.1.1.
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getSpaceSettings
(spaceId)¶ Get space-specific settings.
New in version 4.1.2.
Parameters: spaceId (int) – The Space id. Return type: dict
-
getSpaceStatistics
(id)¶ Returns Space statistics
New in version 4.1.1.
Parameters: id (int) – The Id of the Space
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getSpaces
(sync=None)¶ Returns a list of all known Spaces.
Note
As this function was added with TeamDrive 4, the fall back for TeamDrive 3 is to iterate over all Space Ids, therefore this function is much slower.
See also
getSpaceStatistics
for statistics.returns: A list of Space objects, for example [ { "name": "My Space", "creator": "$TMDR-1007", "icon": "archived", "status": "archived", "status_message": "Space Archived", "id": 1, "spaceRoot": "/home/teamdrive/Spaces/My Space", "time": "2015-09-15T11:17:48Z", "permissionLevel": "read" }, { "name": "Api", "creator": "...", "spaceRoot": "/home/teamdrive/Spaces/Api", "icon": "space", "status": "active", "status_message": "OK", "id": 8, "fileId": 1, "time": "2015-09-15T11:17:48Z", "permissionLevel": "readWrite" } ]
New in version 4.0.
Parameters: sync – (Optional, default
False
) If true, and the key repository has not been fetched from the server yet, wait for the key repository before returningReturn type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getVersion
(versionId)¶ Returns a single version.
>>> versionId = 42 >>> TeamDriveApi().getVersion(versionId)["versionId"] == versionId
New in version 4.1.4.
Parameters: versionId (int) – the id of the version
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
getVersions
(fileId)¶ Returns all versions of a file.
New in version 4.1.4.
Return type: list[dict]
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
importSpaceKeys
()¶ Import Space keys from the server (ie. Spaces that have been deleted locally will re-appear as inactive Spaces, if the key-repository backup was enabled)
Returns: A dict where the key “spacesImported” contains the number of imported Spaces. New in version 4.3.1.
Return type: dict
-
inviteMember
(spaceId, addressId, text, permissionLevel=None, password=None, name=None, sendEmail=True)¶ Invites a user into a Space. If no addressId is given, and ‘name’ is set to the email address of someone who doesn’t have an account yet, they will get an email inviting them to join TeamDrive.
Using this function requires the HTTP POST method.
Throws
TeamDriveException
, if the call fails.Parameters: - spaceId (int) – The Id of the Space
- addressId (int | None) – Already known Addressbook Id (optional if a name is provided)
- text (str) – Invitation Text
- name (str | None) – Username or an email address (required if no addressId is given)
- permissionLevel (str | None) –
The new user permission Level. Optional.
New in version 4.1.1.
- password (str | None) –
Optional. The password for the invitation.
New in version 4.1.1.
- sendEmail (bool | None) –
Optional. If
False
, don’t email the invited user about the invitation (True
by default).New in version 4.5.1.
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Inviting that member failed
- ValueError – no JSON returned. Wrong URL?
-
joinSpace
(id, disableFileSystem, spacePath=None, password=None, backupPath=None, fullRestore=None, snapshotId=None, sync=None, mountInVirtualFS=None)¶ Accepts an invitation, joins an archived Space or rejoins an active one.
- Note: Password protected Invitations:
- Invitations may be protected by a password and accepting these invitations require the password
parameter.If an invitation is password protected,
getSpace
adds"isPasswordProtected": true
to the reply.
Using this function requires the HTTP POST method.
Throws
TeamDriveException
, if the call fails.Parameters: - id (int) – The Id of the Space
- disableFileSystem (bool) – This will disable the synchronization into the file system. TeamDrive will only synchronize meta-data if this is True.
- spacePath (str | None) – Optional, Space root in the file system (must be absolute, to specify a foldername for the Space, use something like /users/bob/MySpace, otherwise use /users/bob/).
- password (str | None) –
Optional, Password for accepting this invitation.
New in version 4.1.1.
- backupPath (str) –
An optional backup path in case the space already exists in the file system.
New in version 4.2.1.
- fullRestore (bool) –
Do a full restore of the space.
New in version 4.2.1.
- snapshotId (int) –
Do a preview-mode rollback of the Space to this snapshot (see
commitCurrentSnapshot
)New in version 4.3.2.
- sync (bool) –
Only return once the Space is active (this can take some time) (default is
False
)New in version 4.5.6.
- mountInVirtualFS (bool) –
Whether to mount the space in the virtual file system.
New in version 4.6.7.
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Joining failed
- ValueError – no JSON returned. Wrong URL?
-
kickMember
(spaceId, addressId)¶ Removes a member form this Space.
Removing a member from a Space will not remove this user from
getSpaceMemberIds
, but will set a this member to status “kicked” ingetMember
.Using this function requires the HTTP POST method.
Throws
TeamDriveException
, if the call fails. For example, if the one doesn’t have the rights to remove a userNew in version 4.0.
Parameters: - spaceId (int) – The Id of the Space
- addressId (int) – Already known Addressbook Id.
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Removing this user failed
- ValueError – no JSON returned. Wrong URL?
-
lockFile
(fileId)¶ Lock a file. Throws
TeamDriveException
, if the call fails.New in version 4.2.1.
Parameters: fileId (int) – The Id of the File
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Failed to queue command.
- ValueError – no JSON returned. Wrong URL?
-
login
()¶ A TeamDrive Client installation is bound to a single TeamDrive account. Calling
login
authenticates TeamDrive user and creates a new device. this is typically the first call to an Agent.Using this function requires the HTTP POST method.
Throws
TeamDriveException
, if the call fails.Example as Command line:
./TeamDriveApi.py '127.0.0.1:45454' --user myUser --pass myPass login
Example with Curl:
curl 'https://127.0.0.1:45454/login' --data-binary '{"username":"My User","password":"My Password"}'
Example:
>>> api = TeamDriveApi("127.0.0.1:45454", "My Username", "My Password") >>> api.login()
Note
On the desktop client, this call is not available, as the API will not be available until the user has successfully logged in. This is a technical limitation of the Gui Client.
See also
Chapter Authentication regarding Authentication.
Parameters: - username (str) – The Username
- password (str) – The Password
- tempPassword (str) – A temporary password, in case of login from a lost-password procedure
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Logging failed.
- ValueError – no JSON returned. Wrong URL?
-
moveFile
(spaceId, *args)¶ Either moveFile(self, spaceId, filePath, trashed, newFilePath) or moveFile(self, spaceId, fileId, newFilePath) Moves a file from filePath or fileId to newFilePath in the given Space.
Using this function requires the HTTP POST method.
Throws
TeamDriveException
, if the call fails.Parameters: - spaceId (int) – The Id of the Space
- filePath (str) – the path of the file in the Space.
- trashed (int) – Is the file in the trash?
- fileId – fileIf of the file meing moved
- newFilePath (str) – The destination file path.
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Failed to queue command.
- ValueError – no JSON returned. Wrong URL?
-
performExternalAuthentication
(authToken)¶ Login using an external authentication token. The registration server must be configured to validate the token, refer the chapter “External Authentication” in the “TeamDrive Registration Server Reference Guide” for details.
Parameters: authToken (str) – The token generated by the authentication service
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Login failed.
-
preLogin
(username)¶ Depending on configuration, some users may need to log in via an external authentication mechanism. This function can be used to check for any such settings before proceeding to login with the user.
Parameters: username (str) – Username or email address Return type: dict
-
publishFile
(fileId, password=None, expiryDate=None, createShortUrl=None, encrypted=None, publishAs=None, versionId=None)¶ Publishes the recent version of that file. The parameters password and expiryDate are optional. Throws
TeamDriveException
, if the call fails.New in version 4.1.
Parameters: - fileId (int) – The Id of the File
- password (str) – If set, people need to enter this password to download the file
- expiryDate (str) – ISO 8601 extended format: either YYYY-MM-DD for dates or YYYY-MM-DDTHH:mm:ss, YYYY-MM-DDTHH:mm:ssTZD (e.g., 1997-07-16T19:20:30+01:00) for combined dates and times.
- createShortUrl (bool | None) –
Whether to create a shortened URL
New in version 4.6.3.
- encrypted (bool | None) –
Enable server-side encryption of the published file
New in version 4.6.3.
- publishAs (str) – Publish the file under a different name
- versionId (int) – Version ID, if publishing a specific file version
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Failed to queue command.
- ValueError – no JSON returned. Wrong URL?
-
putFile
(spaceId, path, data)¶ This is a Python specific accessor to the “files” API. The files API is another API endpoint to handle file content instead of meta data. Urls used by the files API are generated by concatenating the API endpoint
files/
plus the local Space Id (eg.3
) plus the full file path relative to the Space root (eg./my/path/to/the/file
). Such a url could look like this:http://127.0.0.1:45454/files/3/my/path/to/the/file
. This API does not support reading and writing files that are in the trash.This Uploads a file into a Space. The file creation is done asynchronously, a successful return code does not indicate a successful upload to the Server. Use
getFiles
to request the current state of that file.Example using telnet:
telnet 127.0.0.1 45454 PUT /files/3/testfile HTTP/1.1 Host: 127.0.0.1:45454 Accept-Encoding: identity Content-Length: 4 test
Example using curl:
echo "test" | curl -X PUT -d @- http://127.0.0.1:45454/files/3/testfile
Returns: a dict containgng a File object { "file": { "confirmed": false, "creator": "local", "currentVersionId": 6156, "hasComments": false, "icon": "default", "id": 7195, "isDir": false, "name": "testfile", "path": "/", "permissions": "rw-rw-r--", "size": 4, "spaceId": 3, "time": "2015-10-22T14:09:45" }, "result": true }
Note
In TeamDrive 3, this API endpoint was called
/webdav
.Parameters: - spaceId (int) – The Id of the Space
- path (str) – the path of the file in the Space.
- data – The binary data
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
-
putFileContent
(spaceId, spacePath, filePath)¶ Note
This is not part of the HTTP API. See
putFile
instead.Example:
$ python ./TeamDriveApi.py '127.0.0.1:45454' putFileContent 3 /testfile ./testfile
Parameters: - spaceId (int) – The Id of the Space
- spacePath (str) – the path of the file in the Space.
- filePath (str) – the path to the local file
Return type: dict
Raises: httplib.HTTPException – a Connection Error.
-
quit
(logout)¶ Quits TeamDrive.
Using this function requires the HTTP POST method.
Throws
TeamDriveException
, if the call fails.Parameters: logout (bool) – Quit and also log out the current user. See
login
to log in afterwards.Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
removeComment
(commentId)¶ Marks this comment as “removed”
Using this function requires the HTTP POST method.
See also
getComments
,getSpaceComments
,addComment
andaddSpaceComment
New in version 4.1.1.
Parameters: commentId (int) – The id of the comment that will be deleted.
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Removing this comment failed
- ValueError – no JSON returned. Wrong URL?
-
removeLocallyFile
(id, recursive=False)¶ Removes a file form the local file system by disabling the offline-available flag, but does not move this file into the trash or removes the file from the Space. This operation is not synchronized to other clients.
A successful return code does not indicate a successful execution, because it is done asynchronously.
Using this function requires the HTTP POST method.
See also
Parameters: - id (int) – the file to be removed
- recursive (bool) – Directories only: recursive remove
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Failed to queue command.
- ValueError – no JSON returned. Wrong URL?
-
requestActivationEmail
()¶ Request an activation-link email from the regserver for the locally logged in (but not activated) user.
Return type: dict
-
requestResetPassword
(username)¶ This call will reset the password of the user.
New in version 4.1.1.
Parameters: username (str) – The Username
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Logging failed.
- ValueError – no JSON returned. Wrong URL?
-
resolveVersionConflict
(versionId)¶ Changes the current version of a file to this version and resolves all version conflicts on this file.
Using this function requires the HTTP POST method.
Throws
TeamDriveException
, if the call fails.See also
New in version 4.1.4.
Parameters: versionId (int) – The Id of the version
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Failed to queue command.
- ValueError – no JSON returned. Wrong URL?
-
restoreLocallyFile
(id, recursive=False)¶ Restores a file form the local file system. A successful return code does not indicate a successful execution, because it is done asynchronously. This operation is not synchronized to other clients.
Using this function requires the HTTP POST method.
Parameters: - id (int) – the file to be restored
- recursive (bool) – Directories only: recursive restore
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Failed to queue command.
- ValueError – no JSON returned. Wrong URL?
-
sendSupportRequest
(contactName, contactEmail, contactTel, description, sendLogs)¶ Uploads a support request to the server (see the
Client Log Files
chapter in the Registration server documentation on how to configure log uploads)New in version 4.5.0.
Parameters: - contactName (str | None) –
- contactEmail (str | None) –
- contactTel (str | None) –
- description (str | None) – Description of the problem
- sendLogs (bool | None) – Whether or not to attach log files (true if not specified)
Return type: dict
-
setAccountEmail
(email)¶ Sets the account e-mail.
Using this function requires the HTTP POST method.
Returns True, if the license key was successfully changed otherwise raises
TeamDriveException
ExceptionSee also
setLicenseKey
to set the license key.New in version 4.1.1.
Parameters: email (str) – The new e-mail address
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
- TeamDriveException – Failed to set email.
-
setAutoDelete
(fileId, autoDelete)¶ In ‘Data Retention’ Spaces (see Data Retention) with the “Auto Trash” option enabled, files are automatically moved to the trash after their retention period expires. This can be disabled per-file by setting
autoDelete
toFalse
with this call.New in version 4.6.3.
Parameters: - fileId (int) – The id of the file
- autoDelete (bool) – Whether to auto-trash the file
Return type: dict
-
setCurrentVersion
(versionId)¶ Changes the current version of a file to this version.
Using this function requires the HTTP POST method.
Throws
TeamDriveException
, if the call fails.See also
New in version 4.1.4.
Parameters: versionId (int) – The Id of the version
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Failed to queue command.
- ValueError – no JSON returned. Wrong URL?
-
setFeatureChoice
(featureId, useNewFeature, spaceId=0)¶ Set whether the given feature should be used in this Space (ie. suppresses further
Error_Feature_Upgrade
responses for this Space and feature)See also
Chapter
Error_Feature_Upgrade
Note that a response with
useNewFeature == False
will only suppress further error responses for features that have an older behaviour to fall back to.New in version 4.6.3.
Parameters: - featureId (int) – The id of the feature
- useNewFeature (bool) – Whether to use the new feature
- spaceId (int | None) – (Optional, depending on the feature) The Space that this choice is being made for
Return type: dict
-
setLastViewTime
(id)¶ Marks the comments for the given Space as ‘read’.
Parameters: spaceId (int) – Id of the Space Return type: dict
-
setLicenseKey
(licenseKey)¶ Overwrite the license key of this agent.
Using this function requires the HTTP POST method.
Returns True, if the license key was successfully changed otherwise raises
TeamDriveException
ExceptionSee also
setAccountEmail
to set the registration e-mail address.getLicense
to retrieve license infos.Parameters: licenseKey (str) – The new license key
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
setLocalEncryptionEnabled
(enabled)¶ Enable/disable the encryption of local files.
Parameters: enabled (bool) – Whether or not to enable the encryption of all local files. Return type: dict
-
setMemberPermissionLevel
(spaceId, addressId, permissionLevel)¶ Changes the permission level of another member.
Using this function requires the HTTP POST method.
Throws
TeamDriveException
, if the call fails. For example, if the one doesn’t have the rights to remove a userNew in version 4.1.1.
Parameters: - spaceId (int) – The Id of the Space
- addressId (int) – Already known Addressbook Id.
- permissionLevel (str) – the new permission level
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Removing this user failed
- ValueError – no JSON returned. Wrong URL?
-
setProfileInfo
(email=None, phone=None, mobile=None, fullName=None, miscellaneous=None)¶ Sets profile information of the current user.
See also
setProfilePicture
to set the profile picture.New in version 4.1.1.
Parameters: - email (str | None) – Optional, the new e-mail.
- phone (str | None) – Optional, the new phone number.
- mobile (str | None) – Optional, the new mobile number.
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
setProfilePicture
(data)¶ Sets the profile picture of the current user.
See also
getProfilePicture
to retrieve profile pictures andclearProfilePicture
to clear the picture.New in version 4.1.1.
Parameters: data (bytes) – The blob.
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
setProxyCredentials
(username, password)¶ Set authentication credentials for the configured proxy server.
:param username :type username: str :param password :type password: str :rtype: dict
-
setProxySettings
(type, host, port, username, pasword, pacFile)¶ Configure a proxy service for TeamDrive.
Parameters: - type (str) – One of
none
,system
,manual
, orautomatic
- host (str) – Proxy hostname
- port (int) – Port
- username (str) –
- pasword (str) –
- pacFile (str) – Proxy Configuration URI
Return type: dict
- type (str) – One of
-
setRetentionStartTime
(fileId, startTime)¶ In ‘Data Retention’ Spaces (see Data Retention), files are supposed to be un-deletable for a given minimum period of time (the “retention period”). In some cases, however, the actual legal retention period for a file may already have begun before the Space was created -> in such case, this API call can be used to indicate the date at which the retention period for this file actually started.
New in version 4.6.3.
Parameters: - fileId (int) – The id of the file
- startTime (str) – Date from which the retention period for the file should begin (YYYY-MM-DD)
Return type: dict
-
setSetting
(key, value)¶ Set a Setting. must be a valid key as in
getSettings
. An incomplete list of settings can be found in the Options Section.Using this function requires the HTTP POST method.
Parameters: - key (str) – The name of the setting
- value – The new value of the setting
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
setSpacePath
(id, path)¶ Set the root path for a Space in the local file system. This can be used to move a Space to a new location, or to resolve a “Space root not found error” (eg. when a local folder name has changed and TeamDrive can no longer find the Space).
Note
Because this function affects the file system, it can only be called from the host that the API is running on (calling it from elsewhere will result in an “Unknown function” error).
Using this function requires the HTTP POST method.
New in version 4.5.0.
Parameters: - spaceId (int) – The Space id.
- localPath (str) – New root folder for the Space
Return type: dict
-
setSpaceSetting
(spaceId, key, value)¶ set a Space Setting. must be a valid key as in
getSpaceSettings
.Using this function requires the HTTP POST method.
New in version 4.1.2.
Parameters: - spaceId (int) – The Space id.
- key (str) – The name of the setting
- value – The new value of the setting
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
setSpaceSyncEnabled
(id, enabled)¶ Enable/disable synchronization for a Space
Using this function requires the HTTP POST method.
New in version 4.5.0.
Parameters: - spaceId (int) – The Space id.
- enabled (bool) – Whether to enable synchronization
Return type: dict
-
setTrashed
(fileId, inTrash)¶ Moves a file into or out of the trash. Trashing a file also removes the file from the local file system.
Throws
TeamDriveException
, if the call fails.Call
deleteFileFromTrash
to delete the file physically afterwards.See also
New in version 4.0.
Parameters: - fileId (int) – The Id of the Space
- inTrash (bool) – Is the file in the trash?
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Failed to queue command.
- ValueError – no JSON returned. Wrong URL?
-
sse
()¶ This is the Server Side Events api listening on /sse. This API sends events according to the Server Side Events specification.
The implementation of the python wrapper requires Python 3.
Example Usage:
$ python3 TeamDriveApi.py '127.0.0.1:45454' sse data: { "event" : "FileEntryChanged", "fileId" : 0, "hasConflict" : 0, "isDir" : false, "isTrashed" : false, "name" : "my file", "path" : "/home/teamdrive/Spaces/My Space", "spaceId" : 3, "synced" : true } data: { "event" : "SpaceModified", "spaceId" : 3 }
Possible Event types are: FileEntryChanged, VersionEntryChanged, FileVersionPublished, FileVersionUnpublished and SpaceModified
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- ValueError – no JSON returned. Wrong URL?
-
tempPasswordLogin
(username, tempPassword, password, verifyPassword, requireSuperPinConfirm=None, pin=None)¶ Login from a “forgot password” procedure using a temporary password, changing the account password in the process,
Parameters: - username (str) –
- tempPassword (str) – The temporary password
- pasword (str) – The new account password
- verifyPassword (str) – Repeat of the new account password
- requireSuperPinConfirm (bool) – If set to
true
, and if logging in would activate the “Super PIN” functionality for this account (due to local encryption settings), then require an additional confirmation. - pin (str) – If enabled, an existing installation can be logged in to by providing the local pin instead of the account password.
Return type: dict
-
unlockFile
(fileId)¶ Lock a file. Throws
TeamDriveException
, if the call fails.New in version 4.2.1.
Parameters: fileId (int) – The Id of the File
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Failed to queue command.
- ValueError – no JSON returned. Wrong URL?
-
unpublishFile
(fileId, versionId=None)¶ Unpublishes the file. Throws
TeamDriveException
, if the call fails.New in version 4.1.
Parameters: - fileId (int) – The Id of the File
- versionId (int) – Version ID, if unpublishing a specific file version
Return type: dict
Raises: - httplib.HTTPException – a Connection Error.
- socket.error – a Connection Error.
- ConnectionRefusedError – a Connection Error.
- TeamDriveException – Failed to queue command.
- ValueError – no JSON returned. Wrong URL?
-
unsetFeatureChoice
(featureId, spaceId)¶ Cause
Error_Feature_Upgrade
responses for this Space and feature to be shown even if they were suppressed by a previoussetFeatureChoice
call.See also
Chapter
Error_Feature_Upgrade
New in version 4.6.3.
Parameters: - featureId (int) – The id of the feature
- spaceId (int | None) – (Optional, depending on the feature) The Space that this choice is being made for
Return type: dict
-
updateInbox
(id, expiryTime=None, password=None, description=None, maxFileCount=None, maxFileCountInterval=None, maxFileSize=None, maxSize=None)¶ Update the configuration of an existing inbox.
Parameters: - id (int) – The id of the Inbox
- expiryTime – See
createInbox
- password – See
createInbox
- description – See
createInbox
- maxFileCount – See
createInbox
- maxFileCountInterval – See
createInbox
- maxFileSize – See
createInbox
- maxSize – See
createInbox
Return type: dict
-
zipCancel
(spaceId, path)¶ Cancel/clean up a zip-folder task, see
zipFolder
Note
This is not an API function, just a python wrapper for the ‘zip’ endpoint, see
zipFolder
New in version 4.5.0.
Parameters: - spaceId (int) – The Id of the Space
- path (str) – the path of the folder in the Space.
Return type: dict
-
zipDownload
(spaceId, path)¶ Download the result archive of a zip-folder task, see
zipFolder
Note
This is not an API function, just a python wrapper for the ‘zip’ endpoint, see
zipFolder
New in version 4.5.0.
Parameters: - spaceId (int) – The Id of the Space
- path (str) – the path of the folder in the Space.
-
zipFolder
(spaceId, path, recursive)¶ This is a Python specific accessor to the “zip” API which can be used to download a .zip archive of a folder. Because downloading, zipping, and returning the archive can take a long time depending on the size of the folder, this process is broken up into several steps:
- POST to
<api endpoint>/zip[Recursive]/<space id><folder path>
This will start the process of downloading the neccessary files and zipping them. As indicated above, use
.../zipRecursive/...
to include subfolders in the archive, or.../zip/...
to only include the top level
- POST to
- GET to
<api endpoint>/zipProgress/<space id><folder path>
Use this request to poll the status of the task. Returns a json object like:
{ "totalFiles": 100, "filesZipped": 50, "status": "status text", "isDone": false }
- GET to
- GET
<api endpoint>/zip/<space id><folder path>
Downloads the finished archive
- GET
- DELETE
<api endpoint>/zip/<space id><folder path>
This will clean up resources for this job on the server, and cancel the job if is still running.
- DELETE
New in version 4.5.0.
Parameters: - spaceId (int) – The Id of the Space
- path (str) – the path of the folder in the Space.
- recursive (bool) – whether to recursively zip subfolders
Return type: dict
-
zipProgress
(spaceId, path)¶ Get the status of a running zip-folder task, see
zipFolder
Note
This is not an API function, just a python wrapper for the ‘zip’ endpoint, see
zipFolder
New in version 4.5.0.
Parameters: - spaceId (int) – The Id of the Space
- path (str) – the path of the folder in the Space.
Return type: dict
TeamDriveApi.TeamDriveException¶
-
class
TeamDriveApi.
TeamDriveException
(name, result)¶ In case of an error, the TeamDrive API returns a specific JSON document. Thy python Module will parse this error.
Example using curl:
curl -s '127.0.0.1:45454/api/getSpaces' { "error": 50, "error_message": "CJsonApi::ApiError (Error_Missing_Session)", "result": false, "status_code": 500 }
Example using the command line:
$ ./TeamDriveApi.py '127.0.0.1:45454' getSpaces Traceback (most recent call last): File "./TeamDriveApi.py", line 1178, in <module> main() File "./TeamDriveApi.py", line 1174, in main result = (getattr(TeamDriveApi, command)(api, *params)) File "./TeamDriveApi.py", line 307, in getSpaces return self._call("getSpaces") File "./TeamDriveApi.py", line 204, in _call return self._call(name, params, method) File "./TeamDriveApi.py", line 205, in _call raise TeamDriveException(name, jsonRes) __main__.TeamDriveException: Call getSpaces failed with {u'status_code': 500, u'error_message': u'CJsonApi::ApiError (Error_Missing_Session)', u'result': False, u'error': 50}
-
Error_Authentication_Required
= 55¶ This code is returned if a function requires that the user have entered his password for the current API session. An example where this might be returned is when the user has a persistent login to a local installation - if this code is returned, the user must re-login to authenticate himself before performing the API call which returned this code a second time.
-
Error_Confirm_Super_Pin
= 71¶ Returned if proceeding with a login will cause the “Super PIN” functionality to be activated for the user (such as when the installation is set up to encrypt local files), and the
requireSuperPinConfirm
parameter was set to indicate that this activation should require an explicit confirmation step.
-
Error_Feature_Upgrade
= 70¶ The API call would force other members of the Space to upgrade their TeamDrive version, a confirmation step is required via
setFeatureChoice
.In addition to the usual fields, the returned object will include the following:
{ ... "featureId": 10, "featureSpaceId": 123, "featureQuestion": "Using XYZ new feature will require other Space members to upgrade their installation, continue?", "featureHasOldBehaviour": True, ... }
featureId
is the ID of the featurespaceId
is the ID of the Space that this feature-warning applies to (may be omitted for a feature that is not space-specific)featureQuestion
is a prompt that could be displayed to a userfeatureHasOldBehaviour
-> ifTrue
, there is an older behaviour to fall back to, and callingsetFeatureChoice
withFalse
will simply cause further API calls to use the old behaviour
See also
Note
This status is only returned if the setting
http-api-enable-feature-alerts
isTrue
(the default isFalse
)
-
Error_HTTP_Method_Not_Allowed
= 2¶
-
Error_Insufficient_Rights
= 30¶
-
Error_Internal_Error
= 1¶
-
Error_Invalid_File_Key
= 43¶
-
Error_Invalid_Parameter
= 21¶
-
Error_Missing_Parameter
= 20¶
-
Error_Missing_Session
= 50¶ No or invalid credentials supplied.
See also
Chapter Authentication.
-
Error_Unknown_AddressId
= 42¶
-
Error_Unknown_Error
= 3¶
-
Error_Unknown_Space
= 40¶
-
Error_Unknown_User
= 41¶
-
Error_WebportalAccessDisabled
= 33¶ Returned when an attempt is made to access a space from the webportal, when that space has webportal-access disabled
-
Error_Wrong_Login
= 51¶
-
getError
()¶ The TeamDrive API Exception code :rtype: int
-
getErrorString
()¶ A human readable version of this error :rtype: str
-
getStatusCode
()¶ The HTTP status code of this exception. :rtype: int
-
Common JSON Data Types¶
The API returns common data types encoded in JSON objects for different API functions. This section provides an overview of some of these data types.
Space¶
This JSON object describes meta information about a single Space.
Member | Description |
---|---|
id |
Id of the Space. Used to identify a specific Space |
fileId |
FileId of the root-folder in this Space. Only present for active Spaces |
name |
Name of the Space |
spaceRoot |
Local path to the Space. |
invitor |
Invitations only: User name who created the invitation. |
creator |
User name who created the Space |
icon |
Space icon. |
status |
Status of the Space. |
status_message |
Describes the current status of this Space. |
hasTrashedFiles |
Has trashed files in the Space. |
isPasswordProtected |
Invitations only: This invitation needs a password. Obmitted if false. |
time |
Datetime of the last modification of this Space. |
text |
Invitation comment |
permissionLevel |
Permission level of the current user in this Space. (Not for invitations) |
hasComments |
Space has comments. (Not for invitations) |
newComments |
Amount of new comments in the Space. (Not for invitations) |
isOfflineAvailable |
“true”, if the Space will be synchronized to the local file system. TeamDrive will only synchronize meta-data if this is false (Not for invitations) |
Example:
{
"name": "Api",
"creator": "...",
"spaceRoot": "/home/teamdrive/Spaces/Api",
"icon": "space",
"status": "active",
"status_message": "OK",
"id": 8,
"fileId": 1
}
File¶
This JSON object describes meta information about a single File or diretory.
Member | Description |
---|---|
name |
File or Folder Name |
id |
Local Id. Mainly used as parameter. |
spaceId |
Space Id of the file. |
path |
Path to the file excluding the name. |
currentVersionId |
Version id that should be in the file system |
isDir |
Boolean value. true for directories. |
hasNameConflict |
File or Folder has a name conflict |
hasVersionConflict |
File has a version conflict |
permissions |
Unix permissions |
creator |
User name of the file creator. |
created |
file creation time. (Files only) |
icon |
icon name based on the file extension. (Files only) |
confirmed |
The current version is synchronized to the server. (Files only) |
size |
The size in bytes of the current version. (Files only) |
time |
file creation time. (Files only) |
lastModified |
Last modification time. (Files only) |
publicUrl |
URL to the file if it is published. (Files only) |
hasComments |
Boolean value. (Files only) |
isLockedByMe |
Boolean value. (Files only) |
isLockedByOther |
Boolean value. (Files only) |
trasher |
User name who deleted the file. (Files only) |
trashed |
deletion time. (Files only) |
Example:
{
"isDir": true,
"name": "My folder",
"currentVersionId": 0,
"spaceId": 3,
"isInFileSystem": true,
"path": "/",
"id": 756,
"permissions": "rwxr-xr-x"
}
Addressbook Entry¶
This JSON object describes meta information about a addressbook entry.
Member | Description |
---|---|
id |
Addressbook entry Id |
name |
User display name |
email |
Registration Email |
profile |
Profile information |
initials |
User’s initials |
Where Profile information is a JSON object containing:
Member | Description |
---|---|
email |
Display Email |
phone |
Phone number |
mobile |
Mobile nuber |
hasProfilePicture |
Boolean value. true for User’s with profile picture. |